Dependency Injection
What do coffee machines and software architecture have in common? More than you'd think! Both rely on the right components working together seamlessly, and if one part is too tightly coupled to another, everything falls apart.
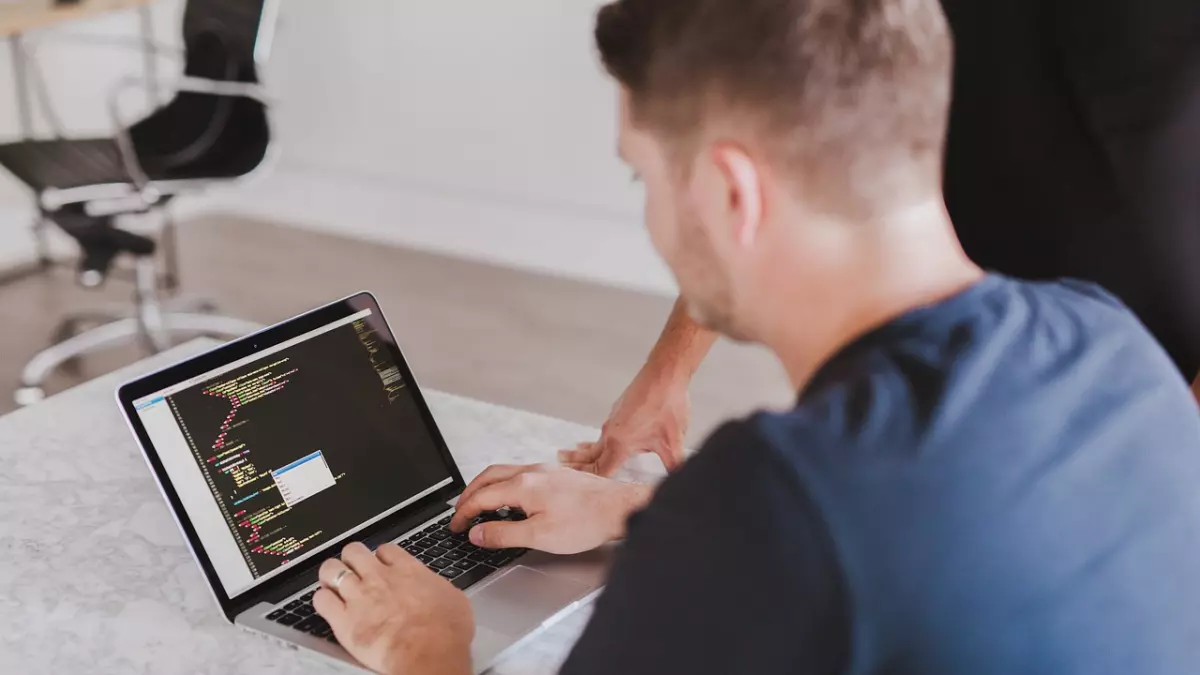
By Carlos Martinez
Dependency Injection (DI) might sound like some fancy term that only enterprise developers need to worry about, but it's actually a game-changer for anyone writing software. Whether you're building a small app or a massive system, mastering DI can make your code more flexible, scalable, and easier to maintain. But let's break it down a bit more, because, like a good cup of coffee, it's all about the right balance.
So, what exactly is Dependency Injection? In simple terms, it's a design pattern that allows a class to receive its dependencies from an external source rather than creating them itself. Think of it like a barista who doesn't grow their own coffee beans but instead gets them from a supplier. The barista can then focus on making the perfect cup of coffee without worrying about where the beans come from.
In software terms, this means that instead of hard-coding dependencies (like services, databases, or other classes) inside your code, you pass them in from the outside. This makes your code more modular and easier to test. Imagine trying to test a coffee machine that grows its own beans—sounds like a nightmare, right? Similarly, tightly coupled code can be a pain to test and maintain.
Why Dependency Injection Matters
Now, you might be thinking, "Okay, but why should I care?" Well, Dependency Injection solves a lot of problems that developers face daily. First off, it makes your code more flexible. When you inject dependencies, you can easily swap them out without changing the core logic of your application. Need to switch from one database to another? No problem. Want to mock a service for testing? Easy peasy.
Another big win is testability. When your code is decoupled, it's much easier to write unit tests. You can inject mock objects or stubs instead of real dependencies, making your tests faster and more reliable. This is a huge advantage in agile development, where continuous testing is key to delivering quality software.
But that's not all. DI also promotes the Single Responsibility Principle (SRP), one of the SOLID principles of object-oriented design. By injecting dependencies, you're ensuring that each class has only one reason to change. It doesn't need to worry about how its dependencies are created or managed; it just uses them. This leads to cleaner, more maintainable code.
How Dependency Injection Works
There are several ways to implement DI, but the most common methods are:
- Constructor Injection: This is the most popular form of DI, where dependencies are passed to a class via its constructor. It's simple, straightforward, and ensures that the class always has its dependencies when it's instantiated.
- Setter Injection: In this approach, dependencies are passed through setter methods. This gives you more flexibility, but it also means that the class might not have all its dependencies when it's created, which can lead to issues.
- Interface Injection: This is a less common method where the class implements an interface that defines a method for injecting dependencies. It's more complex and generally not as widely used as the other two methods.
Most modern frameworks, like Spring for Java or ASP.NET Core for C#, have built-in support for Dependency Injection, making it easier than ever to implement. These frameworks often include Inversion of Control (IoC) containers, which manage the lifecycle of your dependencies and inject them where needed. It's like having a personal assistant who knows exactly what you need and when you need it.
Common Pitfalls of Dependency Injection
Of course, with great power comes great responsibility. While DI can make your code more flexible and testable, it can also lead to some issues if not used correctly. One common pitfall is over-injection, where you inject too many dependencies into a single class. This can make your code harder to understand and maintain. It's like trying to make a coffee with 10 different types of beans—sometimes, less is more.
Another issue is hidden dependencies. If you're not careful, you might end up with dependencies that aren't obvious from the class's interface. This can make your code harder to debug and maintain. To avoid this, always make your dependencies explicit, either through constructors or setters.
Final Thoughts
Dependency Injection isn't just a fancy buzzword—it's a powerful tool that can make your software more flexible, testable, and maintainable. By decoupling your code and injecting dependencies from the outside, you can create systems that are easier to scale and adapt to change. And in today's fast-paced development world, that's a skill every developer should have in their toolkit.
So, the next time you're working on a project, think about how Dependency Injection could make your life easier. After all, who doesn't want their code to be as smooth and reliable as a perfectly brewed cup of coffee?
According to a recent survey, over 70% of developers working in enterprise environments use Dependency Injection in their projects. That’s a pretty strong endorsement!