Code Dependency
Ever had that sinking feeling when you change one tiny thing in your code, and suddenly, everything breaks? Yeah, we’ve all been there. It’s like pulling on a loose thread and watching the whole sweater unravel. The culprit? Software coupling. It’s that invisible web of dependencies that can make your code either a dream to work with or a nightmare to maintain.
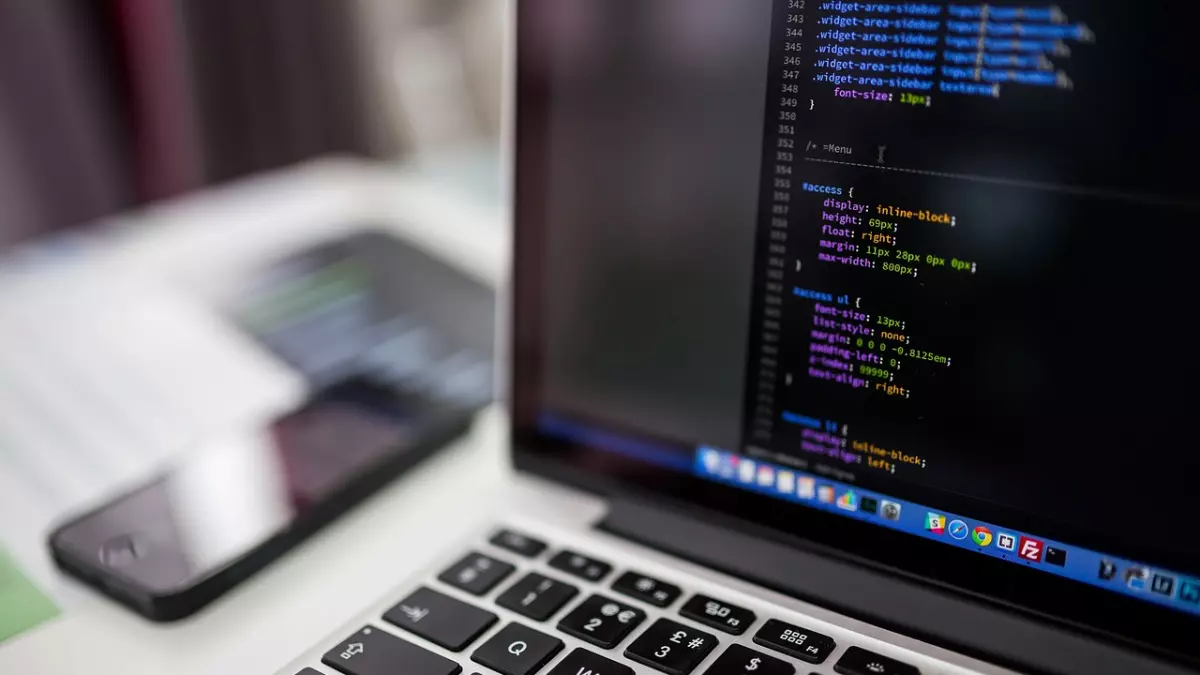
By Mia Johnson
So, what exactly is software coupling? In simple terms, it’s the degree of interdependence between different modules, classes, or components in your software. When two parts of your code are tightly coupled, they rely heavily on each other to function. Change one, and you might have to change the other. On the flip side, loose coupling means that components can operate independently, making your code more flexible and easier to maintain.
But here’s the thing—coupling isn’t inherently bad. In fact, some level of coupling is unavoidable. The real issue arises when your code becomes too tightly coupled, leading to a host of problems like reduced scalability, poor maintainability, and increased technical debt. So, how do you strike the right balance?
The Problem with Tight Coupling
Let’s start with the dark side—tight coupling. Imagine you’re working on a project where every module is so dependent on the others that even a minor change in one part of the system requires you to rewrite half of your codebase. That’s tight coupling in action, and it’s a developer’s worst nightmare.
Why is tight coupling such a big deal? For starters, it makes your code harder to maintain. When everything is interconnected, debugging becomes a Herculean task. You fix one bug, and two more pop up in other parts of the system. It’s like playing a game of whack-a-mole, but with code.
Another issue is scalability. If your code is tightly coupled, scaling your software becomes a monumental challenge. You can’t just add new features or modules without potentially breaking existing functionality. And let’s not even talk about testing—tight coupling makes it nearly impossible to test individual components in isolation, which is a big no-no in modern software development.
Why Loose Coupling is Your Friend
Now, let’s talk about the good stuff—loose coupling. When your code is loosely coupled, each module or component can function independently. This means you can make changes to one part of your system without worrying about breaking everything else. Sounds like a dream, right?
Loose coupling offers several benefits. First, it makes your code more maintainable. Since components are independent, you can easily update or replace them without affecting the rest of your system. This also makes debugging a breeze—you can isolate issues more easily and fix them without causing a ripple effect.
Scalability is another major advantage. When your code is loosely coupled, you can add new features or modules without worrying about breaking existing functionality. This is especially important in today’s fast-paced development environment, where you need to be able to iterate quickly and efficiently.
And let’s not forget about testing. Loose coupling makes it much easier to test individual components in isolation, which is a key principle of unit testing. By reducing dependencies between modules, you can write more effective tests and catch bugs earlier in the development process.
How to Achieve Loose Coupling
Alright, so loose coupling sounds great, but how do you actually achieve it? Here are a few strategies to help you loosen up your code:
- Use Interfaces and Abstractions: One of the best ways to reduce coupling is to introduce interfaces or abstract classes. This allows you to define a contract between components without tying them to specific implementations. By doing so, you can swap out implementations without affecting the rest of your system.
- Follow the SOLID Principles: The SOLID principles are a set of design guidelines that can help you write more maintainable and loosely coupled code. For example, the Dependency Inversion Principle encourages you to depend on abstractions rather than concrete implementations, which can significantly reduce coupling.
- Modularize Your Code: Break your code into smaller, self-contained modules that can function independently. This not only reduces coupling but also makes your code more reusable and easier to test.
- Use Dependency Injection: Dependency injection is a design pattern that allows you to inject dependencies into a class rather than hard-coding them. This makes your code more flexible and easier to test, as you can easily swap out dependencies without modifying the class itself.
- Embrace Event-Driven Architecture: In an event-driven architecture, components communicate through events rather than direct method calls. This decouples them and allows for more flexibility and scalability.
When Coupling is Inevitable
Before you go on a mission to eliminate all coupling from your code, let’s get one thing straight—some level of coupling is inevitable. In fact, certain types of coupling are necessary for your software to function. For example, if two modules need to share data or work together to achieve a common goal, some degree of coupling is required.
The key is to minimize unnecessary coupling and ensure that the coupling that does exist is well-managed. This means using design patterns, abstractions, and other techniques to keep your code as flexible and maintainable as possible.
Final Thoughts
At the end of the day, software coupling is a double-edged sword. Too much of it, and your code becomes a tangled mess that’s impossible to maintain. Too little, and your components might not be able to communicate effectively. The goal is to strike the right balance—minimizing unnecessary dependencies while ensuring that your software remains functional and scalable.
So, the next time you’re writing code, take a step back and think about how tightly coupled your components are. A little bit of planning and foresight can go a long way in making your code more maintainable, scalable, and, ultimately, more enjoyable to work with.