Design Principles
Software development has come a long way since the days of punch cards and assembly language. Back then, the focus was on simply getting the machine to do what you wanted. Today, the game has changed. It's not just about making software work; it's about making it work well, efficiently, and in a way that can be maintained and scaled over time. Enter: software design principles.
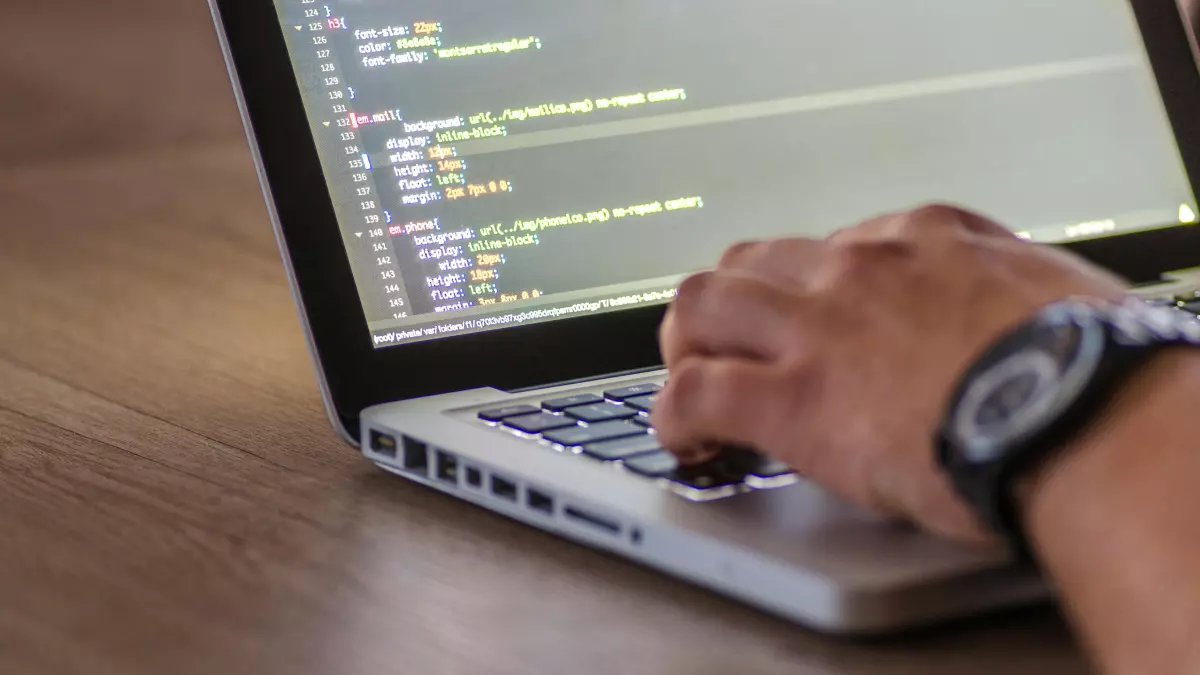
By Kevin Lee
Design principles are the unsung heroes of software development. They’re the guidelines that help developers create software that isn’t just functional but also elegant, scalable, and maintainable. These principles have evolved over time, but their goal remains the same: to make software development less of a headache and more of a joy.
So, what are these magical principles? And why do developers swear by them? Let’s dive into some of the most popular ones that have stood the test of time.
1. DRY (Don't Repeat Yourself)
Ever heard of the phrase, "Work smarter, not harder"? Well, DRY is the software version of that. The idea is simple: avoid duplicating code. If you find yourself writing the same code in multiple places, you're doing it wrong. DRY encourages developers to centralize logic so that if something needs to change, you only have to change it in one place.
Why does this matter? Because code duplication is the enemy of maintainability. Imagine having to update the same logic in 10 different places. Not only is it tedious, but it also increases the chances of making mistakes. DRY makes your codebase cleaner, easier to maintain, and less prone to bugs.
2. KISS (Keep It Simple, Stupid)
Don’t let the name fool you—this principle is anything but stupid. KISS is all about simplicity. The more complex your code, the harder it is to understand, maintain, and debug. The KISS principle reminds developers to avoid over-engineering and to focus on building solutions that are as simple as possible.
It’s easy to get carried away with fancy algorithms and intricate design patterns, but at the end of the day, simpler code is almost always better. It’s easier for other developers (and your future self) to understand, and it’s less likely to break.
3. SOLID Principles
Okay, so this one’s a bit of a cheat because it’s actually five principles rolled into one. But the SOLID principles are so foundational to good software design that they deserve a mention. Here’s a quick breakdown:
- S - Single Responsibility Principle: A class should have one, and only one, reason to change.
- O - Open/Closed Principle: Software entities should be open for extension but closed for modification.
- L - Liskov Substitution Principle: Objects of a superclass should be replaceable with objects of a subclass without affecting the correctness of the program.
- I - Interface Segregation Principle: No client should be forced to depend on methods it does not use.
- D - Dependency Inversion Principle: High-level modules should not depend on low-level modules. Both should depend on abstractions.
These principles are like the Avengers of software design. Each one tackles a specific problem, but together they form a powerful team that can make your code more robust, flexible, and maintainable.
4. YAGNI (You Aren't Gonna Need It)
YAGNI is a principle that’s all about avoiding unnecessary complexity. It’s tempting to build features or write code for hypothetical future scenarios, but YAGNI reminds developers to focus on what’s needed right now. The future is unpredictable, and more often than not, those extra features you thought you’d need never actually get used.
By sticking to YAGNI, you can avoid bloating your codebase with unnecessary functionality, making it easier to maintain and extend in the future.
5. Composition Over Inheritance
Inheritance is one of the cornerstones of object-oriented programming, but it’s not always the best tool for the job. The "Composition Over Inheritance" principle suggests that, instead of using inheritance to share functionality between classes, you should favor composition—building complex objects by combining simpler ones.
Why? Because inheritance can lead to tightly coupled code that’s hard to change. Composition, on the other hand, promotes flexibility and reuse. It allows you to mix and match behaviors without creating a rigid class hierarchy.
6. Law of Demeter (LoD)
Also known as the "Principle of Least Knowledge," the Law of Demeter encourages developers to limit the number of objects that a given object interacts with. In other words, an object should only talk to its immediate friends, not strangers.
This principle helps reduce dependencies between different parts of your code, making it easier to change one part without breaking everything else. It’s all about keeping things modular and decoupled.
So, there you have it—some of the most popular software design principles that developers rely on to create clean, maintainable, and scalable code. These principles might seem simple, but they have a huge impact on the quality of the software you build.
Now, the question is: Are you following these principles in your own projects? If not, maybe it’s time to start!